2. 변환 적용 (RMSE = 23654.49999171906)
2.1 알고리즘을 위한 데이터 준비 및 변환 1~3 적용
우선 훈련할 데이터프레임을 준비한다.
housing = strat_test_set.copy()
여기서 변환 1처럼 housing에 'median_house_value'에서 이상치를 제거한다.
Outlier_line = [112500, 137500, 187500, 225000, 275000, 350000, 450000, 500001]
for i in Outlier_line :
housing = housing[housing.median_house_value != i]
2.1.1 데이터 정제(결측값 제거 or 평균값 적용)
sample_incomplete_rows = housing[housing.isnull().any(axis=1)].head()
sample_incomplete_rows
-118.32 | 34.09 | 44.0 | 2666.0 | NaN | 2297.0 | 726.0 | 1.6760 | 208800.0 | <1H OCEAN |
-117.87 | 33.83 | 27.0 | 2287.0 | NaN | 1140.0 | 351.0 | 5.6163 | 231000.0 | <1H OCEAN |
-118.55 | 34.19 | 18.0 | 5862.0 | NaN | 3161.0 | 1280.0 | 3.1106 | 170600.0 | <1H OCEAN |
-117.02 | 32.78 | 31.0 | 2567.0 | NaN | 1198.0 | 499.0 | 3.4659 | 163000.0 | <1H OCEAN |
-117.92 | 33.70 | 15.0 | 3201.0 | NaN | 1510.0 | 622.0 | 4.2708 | 161700.0 | <1H OCEAN |
sample_incomplete_rows.drop("total_bedrooms", axis=1) # 옵션 2
-118.32 | 34.09 | 44.0 | 2666.0 | 2297.0 | 726.0 | 1.6760 | 208800.0 | <1H OCEAN |
-117.87 | 33.83 | 27.0 | 2287.0 | 1140.0 | 351.0 | 5.6163 | 231000.0 | <1H OCEAN |
-118.55 | 34.19 | 18.0 | 5862.0 | 3161.0 | 1280.0 | 3.1106 | 170600.0 | <1H OCEAN |
-117.02 | 32.78 | 31.0 | 2567.0 | 1198.0 | 499.0 | 3.4659 | 163000.0 | <1H OCEAN |
-117.92 | 33.70 | 15.0 | 3201.0 | 1510.0 | 622.0 | 4.2708 | 161700.0 | <1H OCEAN |
median = housing["total_bedrooms"].median()
sample_incomplete_rows["total_bedrooms"].fillna(median, inplace=True) # 옵션 3
sample_incomplete_rows
-118.32 | 34.09 | 44.0 | 2666.0 | 445.0 | 2297.0 | 726.0 | 1.6760 | 208800.0 | <1H OCEAN |
-117.87 | 33.83 | 27.0 | 2287.0 | 445.0 | 1140.0 | 351.0 | 5.6163 | 231000.0 | <1H OCEAN |
-118.55 | 34.19 | 18.0 | 5862.0 | 445.0 | 3161.0 | 1280.0 | 3.1106 | 170600.0 | <1H OCEAN |
-117.02 | 32.78 | 31.0 | 2567.0 | 445.0 | 1198.0 | 499.0 | 3.4659 | 163000.0 | <1H OCEAN |
-117.92 | 33.70 | 15.0 | 3201.0 | 445.0 | 1510.0 | 622.0 | 4.2708 | 161700.0 | <1H OCEAN |
from sklearn.impute import SimpleImputer
imputer = SimpleImputer(strategy="median")
중간값이 수치형 특성에서만 계산될 수 있기 때문에 텍스트 특성을 삭제합니다:
housing_num = housing.drop("ocean_proximity", axis=1)
# 다른 방법: housing_num = housing.select_dtypes(include=[np.number])
imputer.fit(housing_num)
SimpleImputer(add_indicator=False, copy=True, fill_value=None,
missing_values=nan, strategy='median', verbose=0)
imputer.statistics_
array([-1.1846e+02, 3.4250e+01, 2.8000e+01, 2.1680e+03, 4.4500e+02,
1.1930e+03, 4.1900e+02, 3.4659e+00, 1.7420e+05])
각 특성의 중간 값이 수동으로 계산한 것과 같은지 확인:
housing_num.median().values
array([-1.1846e+02, 3.4250e+01, 2.8000e+01, 2.1680e+03, 4.4500e+02,
1.1930e+03, 4.1900e+02, 3.4659e+00, 1.7420e+05])
훈련 세트를 변환한다.
X = imputer.transform(housing_num)
housing_tr = pd.DataFrame(X, columns=housing_num.columns,
index=housing_num.index)
housing_tr.loc[sample_incomplete_rows.index.values]
-118.32 | 34.09 | 44.0 | 2666.0 | 445.0 | 2297.0 | 726.0 | 1.6760 | 208800.0 |
-117.87 | 33.83 | 27.0 | 2287.0 | 445.0 | 1140.0 | 351.0 | 5.6163 | 231000.0 |
-118.55 | 34.19 | 18.0 | 5862.0 | 445.0 | 3161.0 | 1280.0 | 3.1106 | 170600.0 |
-117.02 | 32.78 | 31.0 | 2567.0 | 445.0 | 1198.0 | 499.0 | 3.4659 | 163000.0 |
-117.92 | 33.70 | 15.0 | 3201.0 | 445.0 | 1510.0 | 622.0 | 4.2708 | 161700.0 |
imputer.strategy
'median'
housing_tr.head()
-117.86 | 33.77 | 39.0 | 4159.0 | 655.0 | 1669.0 | 651.0 | 4.6111 | 240300.0 |
-119.05 | 34.21 | 27.0 | 4357.0 | 926.0 | 2110.0 | 876.0 | 3.0119 | 218200.0 |
-118.15 | 34.20 | 52.0 | 1786.0 | 306.0 | 1018.0 | 322.0 | 4.1518 | 182100.0 |
-117.68 | 34.07 | 32.0 | 1775.0 | 314.0 | 1067.0 | 302.0 | 4.0375 | 121300.0 |
-121.80 | 38.68 | 11.0 | 3851.0 | 892.0 | 1847.0 | 747.0 | 3.4331 | 120600.0 |
2.1.2 텍스트와 범주형 특성 다루기('ocean_proximity' 특성 제거)
이제 범주형 입력 특성인 ocean_proximity을 전처리합니다:
housing_cat = housing[["ocean_proximity"]]
housing_cat.head(10)
<1H OCEAN |
<1H OCEAN |
INLAND |
INLAND |
INLAND |
INLAND |
INLAND |
INLAND |
<1H OCEAN |
INLAND |
from sklearn.preprocessing import OrdinalEncoder
ordinal_encoder = OrdinalEncoder()
housing_cat_encoded = ordinal_encoder.fit_transform(housing_cat)
housing_cat_encoded[:10]
array([[0.],
[0.],
[1.],
[1.],
[1.],
[1.],
[1.],
[1.],
[0.],
[1.]])
ordinal_encoder.categories_
[array(['<1H OCEAN', 'INLAND', 'ISLAND', 'NEAR BAY', 'NEAR OCEAN'],
dtype=object)]
from sklearn.preprocessing import OneHotEncoder
cat_encoder = OneHotEncoder()
housing_cat_1hot = cat_encoder.fit_transform(housing_cat)
housing_cat_1hot
<3825x5 sparse matrix of type ''
with 3825 stored elements in Compressed Sparse Row format>
OneHotEncoder는 기본적으로 희소 행렬을 반환합니다. 필요하면 toarray() 메서드를 사용해 밀집 배열로 변환할 수 있다.
housing_cat_1hot.toarray()
array([[1., 0., 0., 0., 0.],
[1., 0., 0., 0., 0.],
[0., 1., 0., 0., 0.],
...,
[1., 0., 0., 0., 0.],
[0., 1., 0., 0., 0.],
[0., 1., 0., 0., 0.]])
또는 OneHotEncoder를 만들 때 sparse=False로 지정할 수 있다.
cat_encoder = OneHotEncoder(sparse=False)
housing_cat_1hot = cat_encoder.fit_transform(housing_cat)
housing_cat_1hot
array([[1., 0., 0., 0., 0.],
[1., 0., 0., 0., 0.],
[0., 1., 0., 0., 0.],
...,
[1., 0., 0., 0., 0.],
[0., 1., 0., 0., 0.],
[0., 1., 0., 0., 0.]])
cat_encoder.categories_
[array(['<1H OCEAN', 'INLAND', 'ISLAND', 'NEAR BAY', 'NEAR OCEAN'],
dtype=object)]
from sklearn.preprocessing import OneHotEncoder
cat_encoder = OneHotEncoder()
housing_cat_1hot = cat_encoder.fit_transform(housing_cat)
housing_cat_1hot
<3825x5 sparse matrix of type ''
with 3825 stored elements in Compressed Sparse Row format>
#housing = housing.drop("ocean_proximity", axis=1)
import scipy as sp
import numpy as np
one_hot_df = pd.DataFrame(housing_cat_1hot.toarray(), columns=cat_encoder.categories_)
one_hot_df
1.0 | 0.0 | 0.0 | 0.0 | 0.0 |
1.0 | 0.0 | 0.0 | 0.0 | 0.0 |
0.0 | 1.0 | 0.0 | 0.0 | 0.0 |
0.0 | 1.0 | 0.0 | 0.0 | 0.0 |
0.0 | 1.0 | 0.0 | 0.0 | 0.0 |
... | ... | ... | ... | ... |
0.0 | 1.0 | 0.0 | 0.0 | 0.0 |
0.0 | 0.0 | 0.0 | 0.0 | 1.0 |
1.0 | 0.0 | 0.0 | 0.0 | 0.0 |
0.0 | 1.0 | 0.0 | 0.0 | 0.0 |
0.0 | 1.0 | 0.0 | 0.0 | 0.0 |
3825 rows × 5 columns
housing = housing.reset_index(drop=True)
housing = pd.concat([housing, one_hot_df], axis = 1)
housing = housing.drop("ocean_proximity", axis=1)
2.1.3 변환 2, 3
우선 변환 3이 로그함수를 취하는 것이기 때문에 먼저 적용한다.
# 변환 3
housing["total_rooms"] = np.log1p(housing["total_rooms"])
housing["total_bedrooms"] = np.log1p(housing["total_bedrooms"])
housing["population"] = np.log1p(housing["population"])
housing["households"] = np.log1p(housing["households"])
housing["median_income"] = np.log1p(housing["median_income"])
또한, 특성 3개를 추가한다.
housing["rooms_per_household"] = housing["total_rooms"]/housing["households"]
housing["bedrooms_per_room"] = housing["total_bedrooms"]/housing["total_rooms"]
housing["population_per_household"]=housing["population"]/housing["households"]
#특성 3개 추가
이미 범주형을 수치형으로 바꾸고 특성에 적용했기 때문에 변환 2를 적용하면 된다.
# 변환 2
corr_matrix = housing.corr()
corr_matrix2 = abs(corr_matrix["median_house_value"])
delist = []
i = 0
while i < len(housing.columns):
if corr_matrix2[i] < 0.2:
delist.append(housing.columns[i])
i += 1
housing = housing.drop(delist, axis=1)
housing
1.724747 | 240300.0 | 1.0 | 0.0 | 1.145143 |
1.389265 | 218200.0 | 1.0 | 0.0 | 1.129626 |
1.639346 | 182100.0 | 0.0 | 1.0 | 1.198857 |
1.616910 | 121300.0 | 0.0 | 1.0 | 1.220488 |
1.489099 | 120600.0 | 0.0 | 1.0 | 1.136678 |
... | ... | ... | ... | ... |
1.089504 | 76400.0 | 0.0 | 1.0 | 1.115807 |
1.090244 | 134000.0 | 0.0 | 0.0 | 1.176936 |
1.774681 | 311700.0 | 1.0 | 0.0 | 1.118708 |
1.742394 | 133500.0 | 0.0 | 1.0 | 1.156589 |
1.565632 | 78600.0 | 0.0 | 1.0 | 1.191604 |
3825 rows × 5 columns
적용 결과 상관계수가 0.2 이하인 특성은 제거되었다.
마무리로 'median_house_value' 특성을 housing 데이터프레임에서 제거하고 housing_labels에 따로 넣어놓는다.
housing_labels = housing["median_house_value"].copy()
housing = housing.drop("median_house_value", axis=1) # 훈련 세트를 위해 레이블 삭제
2.1.4 변환 파이프라인
수치형 특성을 전처리하기 위해 파이프라인을 만든다.
from sklearn.pipeline import Pipeline
from sklearn.preprocessing import StandardScaler
num_pipeline = Pipeline([
('imputer', SimpleImputer(strategy="median")),
('std_scaler', StandardScaler()),
])
housing_prepared = num_pipeline.fit_transform(housing)
하지만 원본과 다르게 범주형을 미리 수치형으로 바꿔놓아서 0과 1로 이루어져야 할 2, 3번째 열이 1.4와 -0.7로 구성되어 있다.
housing_prepared
array([[ 0.69872277, 1.11744228, -0.70087664, -0.48992832],
[-0.31600474, 1.11744228, -0.70087664, -0.71399503],
[ 0.44041266, -0.89490081, 1.4267846 , 0.28567843],
...,
[ 0.84975854, 1.11744228, -0.70087664, -0.87163833],
[ 0.75210051, -0.89490081, 1.4267846 , -0.324654 ],
[ 0.2174513 , -0.89490081, 1.4267846 , 0.18094796]])
이하 0과 1로 이루어지게 하기 위해서 파이프라인 적용 전 housing에 있는 데이터를 housing_prepared에 적용하는 과정이다.
<1H OCEAN 부터 적용해보자.
ilst = list(np.array(housing.iloc[:,1].tolist()))
ilst2 = []
i = 0
while i < len(ilst) :
ilst2.append([ilst[i]])
i += 1
housing_prepared[:,[1]] = ilst2
반복문을 사용하면 되지만 한번 더 사용해서 INLAND 항목도 바꿔주었다.
ilst = list(np.array(housing.iloc[:,2].tolist()))
ilst2 = []
i = 0
while i < len(ilst) :
ilst2.append([ilst[i]])
i += 1
housing_prepared[:,[2]] = ilst2
성공적으로 적용하였다.
housing_prepared
array([[ 0.69872277, 1. , 0. , -0.48992832],
[-0.31600474, 1. , 0. , -0.71399503],
[ 0.44041266, 0. , 1. , 0.28567843],
...,
[ 0.84975854, 1. , 0. , -0.87163833],
[ 0.75210051, 0. , 1. , -0.324654 ],
[ 0.2174513 , 0. , 1. , 0.18094796]])
housing_prepared.shape
(3825, 4)
2.2 모델 선택과 훈련
모델 선택과 훈련에서 따로 바꿀 내용은 없기 때문에 약간의 수정만 하였다.
ex) 변환 적용에 따른 파이프라인의 transform 항목 제거
from sklearn.model_selection import cross_val_score
scores = cross_val_score(tree_reg, housing_prepared, housing_labels,
scoring="neg_mean_squared_error", cv=10)
tree_rmse_scores = np.sqrt(-scores)
def display_scores(scores):
print("점수:", scores)
print("평균:", scores.mean())
print("표준 편차:", scores.std())
display_scores(tree_rmse_scores)
점수: [77697.36311476 77602.91490717 77140.81018048 79333.33840023
80693.22220398 83968.49306273 82017.56835169 85870.58797738
84456.23677603 79337.3321803 ]
평균: 80811.78671547429
표준 편차: 2968.815172358624
lin_scores = cross_val_score(lin_reg, housing_prepared, housing_labels,
scoring="neg_mean_squared_error", cv=10)
lin_rmse_scores = np.sqrt(-lin_scores)
display_scores(lin_rmse_scores)
점수: [60760.79713215 64794.52182928 60970.09857881 62272.59618758
69197.96529907 65123.8049494 62991.55649597 58848.39293478
65417.32956484 64469.86482141]
평균: 63484.69277932766
표준 편차: 2805.350279527959
from sklearn.ensemble import RandomForestRegressor
forest_reg = RandomForestRegressor(n_estimators=100, random_state=42)
forest_reg.fit(housing_prepared, housing_labels)
RandomForestRegressor(bootstrap=True, ccp_alpha=0.0, criterion='mse',
max_depth=None, max_features='auto', max_leaf_nodes=None,
max_samples=None, min_impurity_decrease=0.0,
min_impurity_split=None, min_samples_leaf=1,
min_samples_split=2, min_weight_fraction_leaf=0.0,
n_estimators=100, n_jobs=None, oob_score=False,
random_state=42, verbose=0, warm_start=False)
housing_predictions = forest_reg.predict(housing_prepared)
forest_mse = mean_squared_error(housing_labels, housing_predictions)
forest_rmse = np.sqrt(forest_mse)
forest_rmse
22925.449436433246
from sklearn.model_selection import cross_val_score
forest_scores = cross_val_score(forest_reg, housing_prepared, housing_labels,
scoring="neg_mean_squared_error", cv=10)
forest_rmse_scores = np.sqrt(-forest_scores)
display_scores(forest_rmse_scores)
점수: [61866.53256173 62373.77008567 60223.17847966 59497.55647123
63792.15328368 60100.00748396 63288.61654002 58050.76324036
63063.16415009 61881.88460613]
평균: 61413.76269025303
표준 편차: 1770.9644804718287
scores = cross_val_score(lin_reg, housing_prepared, housing_labels, scoring="neg_mean_squared_error", cv=10)
pd.Series(np.sqrt(-scores)).describe()
count 10.000000
mean 63484.692779
std 2957.098839
min 58848.392935
25% 61295.722981
50% 63730.710659
75% 65041.484169
max 69197.965299
dtype: float64
from sklearn.svm import SVR
svm_reg = SVR(kernel="linear")
svm_reg.fit(housing_prepared, housing_labels)
housing_predictions = svm_reg.predict(housing_prepared)
svm_mse = mean_squared_error(housing_labels, housing_predictions)
svm_rmse = np.sqrt(svm_mse)
svm_rmse
97387.4906726381
2.3 모델 세부 튜팅
모델 세부 튜닝에서 따로 바꿀 내용은 없기 때문에 약간의 수정만 하였다.
ex) 파라미터 값 설정 변경
2.3.1 그리드 탐색
상관계수가 낮은 특성은 제거하였기 때문에 'max_fatures' 수치를 수정하였다.
from sklearn.model_selection import GridSearchCV
param_grid = [
# 12(=3×4)개의 하이퍼파라미터 조합을 시도합니다.
{'n_estimators': [3, 10, 30], 'max_features': [2, 3, 4]},
# bootstrap은 False로 하고 6(=2×3)개의 조합을 시도합니다.
{'bootstrap': [False], 'n_estimators': [3, 10], 'max_features': [2, 3, 4]},
]
forest_reg = RandomForestRegressor(random_state=42)
# 다섯 개의 폴드로 훈련하면 총 (12+6)*5=90번의 훈련이 일어납니다.
grid_search = GridSearchCV(forest_reg, param_grid, cv=5,
scoring='neg_mean_squared_error',
return_train_score=True)
grid_search.fit(housing, housing_labels)
GridSearchCV(cv=5, error_score=nan,
estimator=RandomForestRegressor(bootstrap=True, ccp_alpha=0.0,
criterion='mse', max_depth=None,
max_features='auto',
max_leaf_nodes=None,
max_samples=None,
min_impurity_decrease=0.0,
min_impurity_split=None,
min_samples_leaf=1,
min_samples_split=2,
min_weight_fraction_leaf=0.0,
n_estimators=100, n_jobs=None,
oob_score=False, random_state=42,
verbose=0, warm_start=False),
iid='deprecated', n_jobs=None,
param_grid=[{'max_features': [2, 3, 4],
'n_estimators': [3, 10, 30]},
{'bootstrap': [False], 'max_features': [2, 3, 4],
'n_estimators': [3, 10]}],
pre_dispatch='2*n_jobs', refit=True, return_train_score=True,
scoring='neg_mean_squared_error', verbose=0)
최상의 파라미터 조합은 다음과 같다.
grid_search.best_params_
{'max_features': 2, 'n_estimators': 30}
grid_search.best_estimator_
RandomForestRegressor(bootstrap=True, ccp_alpha=0.0, criterion='mse',
max_depth=None, max_features=2, max_leaf_nodes=None,
max_samples=None, min_impurity_decrease=0.0,
min_impurity_split=None, min_samples_leaf=1,
min_samples_split=2, min_weight_fraction_leaf=0.0,
n_estimators=30, n_jobs=None, oob_score=False,
random_state=42, verbose=0, warm_start=False)
그리드서치에서 테스트한 하이퍼파라미터 조합의 점수를 확인한다.
cvres = grid_search.cv_results_
for mean_score, params in zip(cvres["mean_test_score"], cvres["params"]):
print(np.sqrt(-mean_score), params)
67889.07359833093 {'max_features': 2, 'n_estimators': 3}
62939.68210829389 {'max_features': 2, 'n_estimators': 10}
61426.63997668008 {'max_features': 2, 'n_estimators': 30}
68658.64272288956 {'max_features': 3, 'n_estimators': 3}
62779.91520182231 {'max_features': 3, 'n_estimators': 10}
61520.99358149143 {'max_features': 3, 'n_estimators': 30}
69075.49784814547 {'max_features': 4, 'n_estimators': 3}
63832.9164379589 {'max_features': 4, 'n_estimators': 10}
61913.367380477204 {'max_features': 4, 'n_estimators': 30}
71432.45751890287 {'bootstrap': False, 'max_features': 2, 'n_estimators': 3}
67329.20010071111 {'bootstrap': False, 'max_features': 2, 'n_estimators': 10}
72564.13705673715 {'bootstrap': False, 'max_features': 3, 'n_estimators': 3}
68869.9072946684 {'bootstrap': False, 'max_features': 3, 'n_estimators': 10}
81041.5454306058 {'bootstrap': False, 'max_features': 4, 'n_estimators': 3}
80576.89294191488 {'bootstrap': False, 'max_features': 4, 'n_estimators': 10}
pd.DataFrame(grid_search.cv_results_)
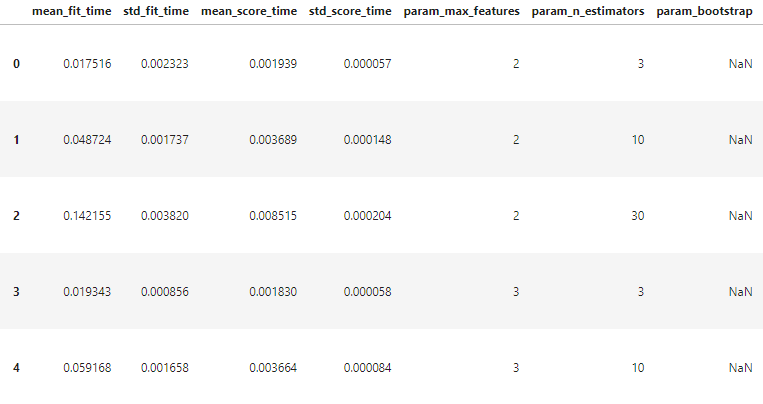
2.3.2 최상의 모델과 오차 분석
feature_importances = grid_search.best_estimator_.feature_importances_
feature_importances
array([0.53994085, 0.03048519, 0.17934518, 0.25022878])
2.3.3 테스트 세트로 시스템 평가하기 (결과)
결과를 확인해보면 rmse = 23654.49999171906 값이 나오는 것을 확인할 수 있다.
final_model = grid_search.best_estimator_
X_test = housing
y_test = housing_labels
final_predictions = final_model.predict(X_test)
final_mse = mean_squared_error(y_test, final_predictions)
final_rmse = np.sqrt(final_mse)
final_rmse
23654.49999171906
테스트 RMSE에 대한 95% 신뢰 구간을 계산할 수 있다.
from scipy import stats
confidence = 0.95
squared_errors = (final_predictions - y_test) ** 2
np.sqrt(stats.t.interval(confidence, len(squared_errors) - 1,
loc=squared_errors.mean(),
scale=stats.sem(squared_errors)))
array([22793.42057051, 24485.31638375])
다음과 같이 수동으로 계산할 수도 있다.
m = len(squared_errors)
mean = squared_errors.mean()
tscore = stats.t.ppf((1 + confidence) / 2, df=m - 1)
tmargin = tscore * squared_errors.std(ddof=1) / np.sqrt(m)
np.sqrt(mean - tmargin), np.sqrt(mean + tmargin)
(22793.42057050913, 24485.316383750298)
요약 정리
'housing' 데이터셋을 활용한 데이터 전처리
- housing 데이터셋 다운로드 후 csv 형식의 파일을 pandas로 확인하는 것으로 시작한다.
- 훈련 세트와 테스트 세트를 구분하여 만들고, 고유 식별자를 이용하여 무작위로 테스트 세트를 만드는 것을 방지한다.
변환 1
- "median_income"과 "median_house_value" 특성의 상관관계 그래프에서 500,000의 값이 집중적으로 존재하는 것을 눈으로도 확인할 수 있다. 이것을 loc을 이용해 내림차순으로 정리하면 그 값이 500,001이라는 것을 확인할 수 있다.
- 존재하는 값을 count해보면 450,000, 350,000 등 수평선을 보이는 수치들을 확인할 수 있는데, 이것을 삭제하여 housing 데이터셋에서 이상치를 제거한다.
변환 2
- housing 데이터셋에는 9개의 특성이 존재하는데 그 중 "rooms_per_household", "bedrooms_per_room", "population_per_household" 특성은 기존 9개의 특성을 조합하여 생성하였다.
- 특성간에 상관계수를 확인해보면 abs 값을 적용시켜보면 0.2보다 낮은 항목이 있다. 더욱 효과적인 학습을 위해 이 특성들을 제거하였다.
변환 3
- 특성들의 히스토그램을 확인하면 비대칭적인 모양의 특성들이 존재한다. numpy의 로그변환을 이용하여 좌우 비대칭 현상을 해결했다.
실제 데이터와 변환 적용한 데이터 비교
원본 데이터셋 - RMSE = 47,730
변환 데이터셋 - RMSE = 23,654
RMSE(Root Mean Square Error): 평균 제곱근 오차, 값은 낮을수록 좋다.
- 원본 데이터셋은 범주형 특성을 one_hot 인코딩으로 수치형 특성으로 변환하고, 테스트 세트를 제작하여 랜덤탐색(RandomizedSearch)을 이용하여 학습하였다.
- 변환 데이터셋은 원본 데이터셋과 마찬가지로 진행하나, 변환 1~3을 적용하였다.
참고 문헌 : 핸즈온 머신러닝
Github 링크: 머신러닝 실습 2